Fraud in financial services is a massive problem. According to NASDAQ, in 2023, banks faced $442 billion in projected losses from payments, checks, and credit card fraud. It’s not just about the money, though. Fraud can tarnish a company’s reputation and frustrate customers when legitimate purchases are blocked. This is called a false positive. Unfortunately, these errors happen more often than you’d think because traditional fraud detection methods simply aren’t keeping up with how sophisticated fraud has become.
This post focuses on credit card transaction fraud, one of the most prevalent forms of financial fraud. While other types of fraud, such as identity theft, account takeover, and money laundering, are also significant concerns, credit card fraud poses a unique challenge due to its high transaction volume and broad attack surface, making it a key target for fraudsters. Financial institutions are estimated to lose $43 billion by 2026 in annual credit card losses, according to Nilson.
Traditional fraud detection methods, which rely on rules-based systems, or statistical methods, are reactive and increasingly ineffective in identifying sophisticated fraudulent activities. As data volumes grow and fraud tactics evolve, financial institutions need more proactive, intelligent approaches to detect and prevent fraudulent transactions.
AI offers essential tools for analyzing vast amounts of transactional data, identifying abnormal behaviors, and recognizing patterns that indicate fraud. But while steps have been taken to improve detection, even more advanced techniques are needed to improve accuracy, reduce false positives, and enhance operational efficiency in fraud detection.
This post introduces an end-to-end AI workflow that uses graph neural networks (GNNs) offering a flexible, high-performance solution for fraud detection. It also walks through how you can get started with model building and inference with this fraud detection workflow.
Graph neural networks for fraud detection
Traditional machine learning (ML) models, such as XGBoost, have been widely used for fraud detection and have proven effective at identifying anomalous behavior in individual transactions. However, fraud detection is rarely a problem of isolated events. Fraudsters operate within complex networks, often using connections between accounts and transactions to hide their activities. This is where GNNs come in.
GNNs are designed to work with graph-structured data, making them particularly suited for fraud detection in financial services. Imagine each account, transaction, and device as a node within a network. Instead of analyzing individual transactions only, GNNs consider the connections between these nodes—revealing patterns of suspicious activity across the network.
For example, suppose an account has a relationship with known fraudulent entities or is similar to other high-risk entities, GNNs can pick up on that connection and flag it for further investigation, even if the account itself seems fine.
Combining GNNs with XGBoost offers the best of both worlds:
- Higher accuracy: GNNs don’t just focus on individual transactions—they consider how everything is connected, catching fraud that might otherwise go undetected.
- Fewer false positives: With more context, GNNs help reduce false alarms, so legitimate transactions don’t get flagged unnecessarily.
- Better scalability: GNNs model building scales to handle massive networks of data efficiently. But combining GNNs with XGBoost real-time fraud detection (inference) is possible even at large scales.
- Explainability: Combining GNNs with XGBoost provides the power of deep learning with the explainability of decision trees.
An end-to-end fraud detection AI workflow using GNNs
NVIDIA has built an end-to-end fraud detection workflow that combines traditional ML with the power of GNNs. This process builds on a standard XGBoost approach but augments it with GNN embeddings to significantly boost accuracy. While exact numbers are confidential, even a small improvement—such as 1%—could translate into millions of dollars in savings, making GNNs a critical part of fraud detection systems.
The general architecture includes two main parts: the model building step and the inference process, as shown in Figure 1.
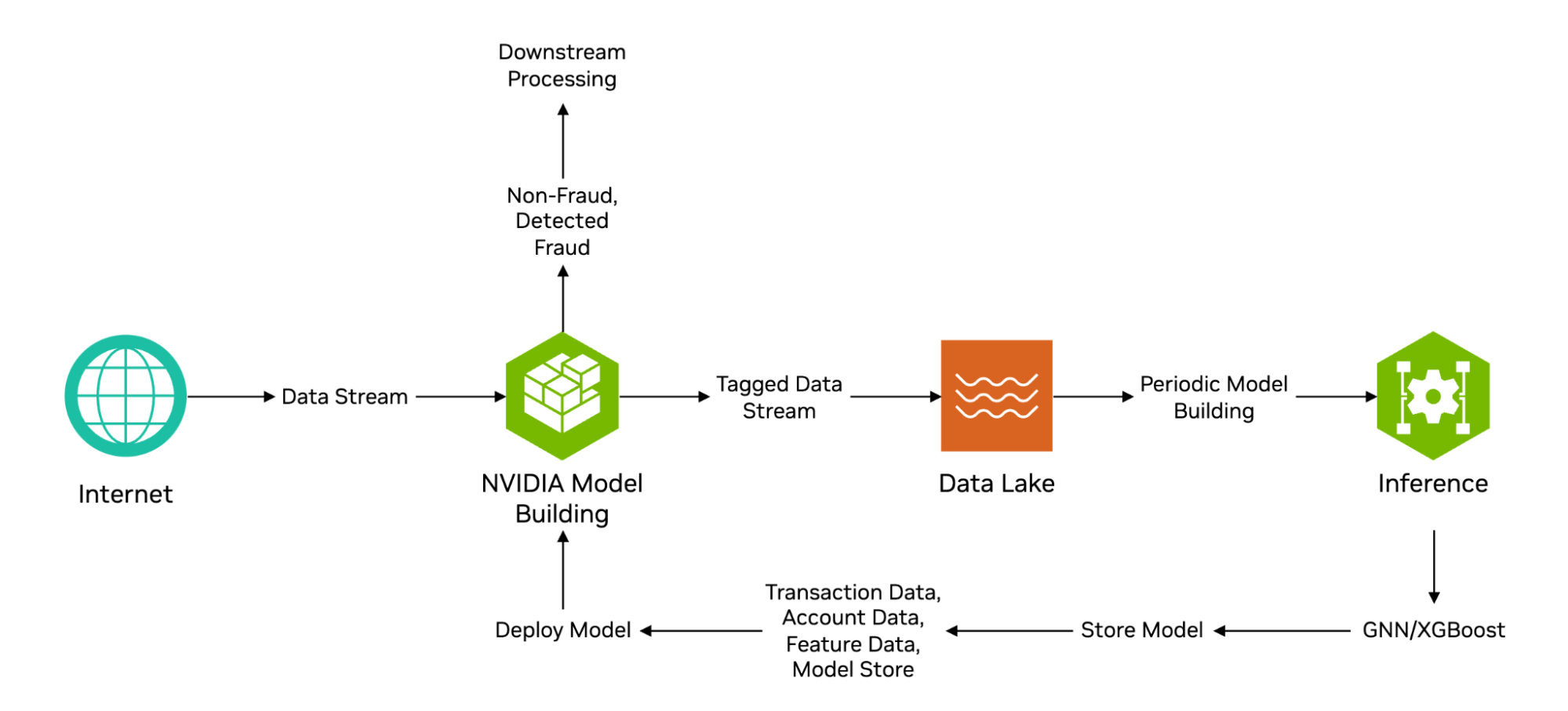
Model building with GNNs and XGBoost
The process starts with the model building phase, since a model needs to be available for inference in the workflow above, where GNNs are used to create features (embeddings) that are fed into an XGBoost model (Figure 2).
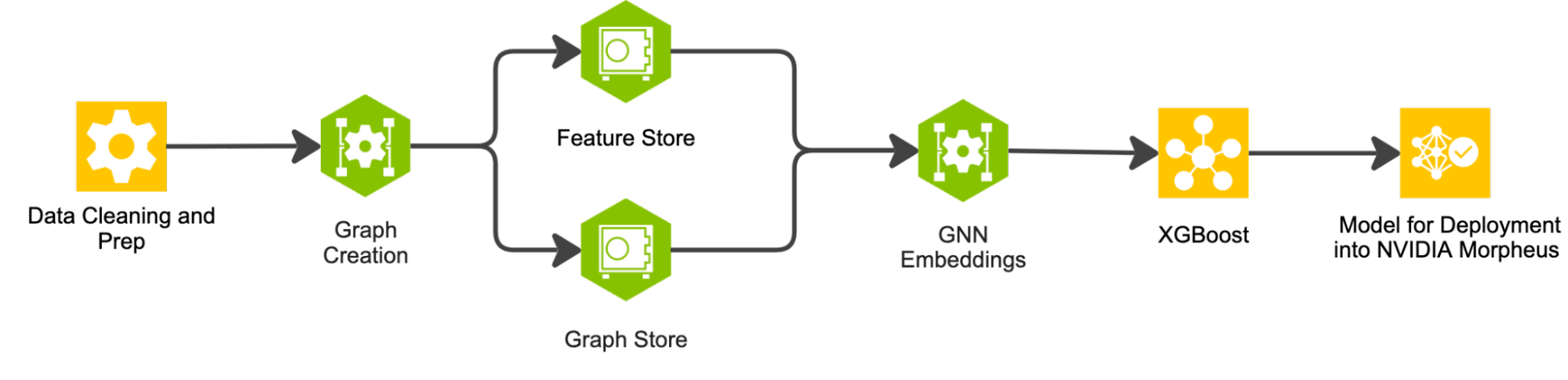
Step 1: Data preparation
Incoming transaction data is cleaned and prepared, typically using tools like RAPIDS for efficiency. Data preparation and feature engineering have a significant impact on the performance of model building. This step requires a detailed understanding of the data and could take multiple tries to get the best results.
Once a script for data preparation has been created, it can be automated in the workflow. The data preparation process should be evaluated as new data is added or periodically as data grows. The next iteration of this workflow will leverage NVIDIA RAPIDS Accelerator for Apache Spark to accelerate the data processing piece of this workflow.
Step 2: Graph creation
For large datasets, typical of FSI, the graph creation process converts the prepared data into a Feature Store (tabular data) and a Graph Store (structural data). This enables better host and device memory usage and peak performance. The two stores are optimized for GNN frameworks like PyG (PyTorch Geometric) and DGL (Deep Graph Library). A key benefit of using this workflow is ensuring the stores are optimized for the selected GNN framework.
# load the edge data
edge_data = cudf.read_csv(edge_path, header=None,
names=[edge_src_col, edge_dst_col, edge_att_col], dtype=['int32','int32','float'])
# convert to tensors
num_nodes = max(edge_data[edge_src_col].max(), edge_data[ edge_dst_col].max()) + 1
src_tensor = torch.as_tensor(edge_data[edge_src_col], device='cuda')
dst_tensor = torch.as_tensor(edge_data[edge_dst_col], device='cuda')
# save in a GraphStore
graph_store = cugraph_pyg.data.GraphStore()
graph_store[("n", "e", "n"), "coo", False, (num_nodes, num_nodes)] = [src_tensor, dst_tensor]
...
# load the features
feature_data = cudf.read_csv(feature_path)
# convert to tensors
col_tensors = []
for c in feature_columns:
t = torch.as_tensor(feature_data[c].values, device='cuda')
col_tensors.append(t)
x_feature_tensor = torch.stack(col_tensors).T
feature_store = cugraph_pyg.data.TensorDictFeatureStore()
feature_store["node", "x"] = x_feature_tensor
feature_store["node", "y"] = y_label_tensor
Step 3: GNN embedding generation
Rather than having the GNN produce a classification, the last layer of the GNN is extracted as embeddings. Those GNN embeddings are passed into XGBoost and a model is created. That model is then saved for use in inferencing.
def extract_embeddings(model, loader):
model.eval()
embeddings = []
labels = []
with torch.no_grad():
for batch in loader:
batch_size = batch.batch_size
hidden = model(batch.x[:,:].to(torch.float32), batch.edge_index, return_hidden=True)[:batch_size]
embeddings.append(hidden) # Keep embeddings on GPU
labels.append(batch.y[:batch_size].view(-1).to(torch.long))
embeddings = torch.cat(embeddings, dim=0) # Concatenate embeddings on GPU
labels = torch.cat(labels, dim=0) # Concatenate labels on GPU
return embeddings, labels
... in main code ....
# Define the model
model = GraphSAGE( ....)
for epoch in range(best_params['num_epochs']):
train_loss = train_gnn(model, train_loader, optimizer, criterion)
...
# Extract embeddings from the second-to-last layer and keep them on GPU
embeddings, labels = extract_embeddings(model, train_loader)
By using GPU-accelerated versions of GNN frameworks—such as cuGraph-pyg and cuGraph-dgl—this workflow can handle large datasets with complex graph structures efficiently.
Inference for real-time fraud detection
Once the model is trained, it can be served for real-time fraud detection using NVIDIA Triton Inference Server, an open-source AI model-serving platform that streamlines and accelerates the deployment of AI inference workloads in production. NVIDIA Triton helps enterprises reduce the complexity of model-serving infrastructure, shorten the time needed to deploy new AI models in production, and increase AI inferencing and prediction capacity.
The trained model can also be deployed using NVIDIA Morpheus, an open-source cybersecurity AI framework that enables developers to create optimized applications for filtering, processing, and classifying large volumes of streaming data. The Morpheus Runtime Core (MRC) orchestrating this workflow accelerates massive data processing and analysis and helps with inferencing by periodically triggering the process to build a new model.
As shown in Figure 3, the inference process involves:
- Transforming the raw input data using the same process used during model building (that is, training).
- Feeding the data into the GNN model to convert the transaction into an embedding. This is needed since the XGBoost model was trained on the embedding.
- Feeding the embeddings into the XGBoost model to predict if the transactions are fraudulent.
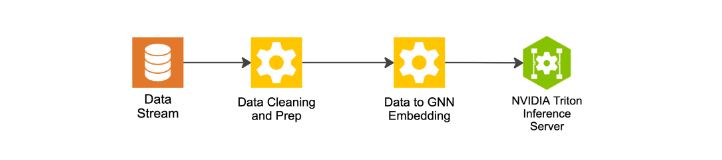
# Load GNN model for creating node embeddings
gnn_model = torch.load(gnn_model_path)
gnn_model.eval() # Set the model to evaluation mode
# Load xgboost model for node classification
loaded_bst = xgb.Booster()
loaded_bst.load_model(xgb_model_path)
# Generate node embedding using the GNN model
transaction_embeddings = gnn_model(X.to(device), ....)
# Convert embeddings to cuDF DataFrame
embeddings_cudf = cudf.DataFrame(cp.from_dlpack(to_dlpack(embeddings)))
# Create DMatrix for the test embeddings
dtest = xgb.DMatrix(embeddings_cudf)
# Predict using XGBoost on GPU
preds = bst.predict(dtest)
By incorporating both GNNs and XGBoost, this AI workflow offers a flexible, high-performance solution for fraud detection. Enterprises can customize the configuration of GNNs and adjust model-building processes based on their unique needs, ensuring the system stays optimized over time.
Ecosystem using the AI workflow to enhance fraud detection
Amazon Web Services (AWS) is the first cloud service provider to integrate this end-to-end fraud detection workflow with their highly secure accelerated computing capabilities. With this simple workflow integration, developers who build fraud detection models can use NVIDIA RAPIDS within Amazon EMR for data processing, leverage RAPIDS and GNN libraries within Amazon SageMaker and Amazon EC2 services for model training. This integration flexibly scales low latency and high throughput predictions using NVIDIA Morpheus and NVIDIA Triton Inference Server through Amazon SageMaker or Amazon Elastic Kubernetes Service endpoint.
As these efforts continue to develop, this workflow will be available across the NVIDIA partner ecosystem for enterprises and developers to prototype and take it to production through NVIDIA AI Enterprise.
Get started
As fraud tactics evolve, traditional detection methods fall short. Combining XGBoost with GNNs offers a powerful solution—boosting accuracy, reducing false positives, and improving real-time detection. This AI workflow is designed to help enterprises stay ahead of sophisticated fraud attempts and adapt quickly to new threats.
To learn more about using GNNs to transform your approach to fraud detection, check out the AI Credit Card Fraud Workflow. You can also explore the NVIDIA LaunchPad lab Deploy a Fraud Detection XGBoost Model with NVIDIA Triton and the AI for Fraud Detection Use Case.